So, after searching the blogosphere, I used info from these two links to accomplish what we needed:
- CRMService.Retrieve Method using JScript (MSDN article)
- Richard Knudson's Dynamics CRM Trick Bag - Useful Dynamics CRM Form Scripts #3
To do this fix, follow these steps:
- In the Case entity, add a new Attribute called Related Account (or similar). Be sure to note the Name (case_relatedaccount in this case) as this is used in the JScript.
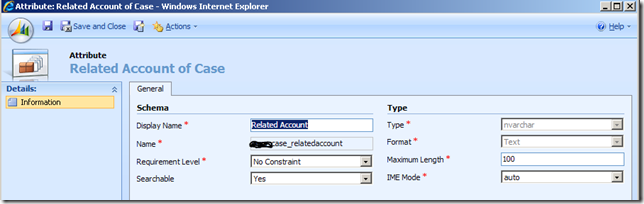
- Save and Close this, and add the attribute to the Case form.
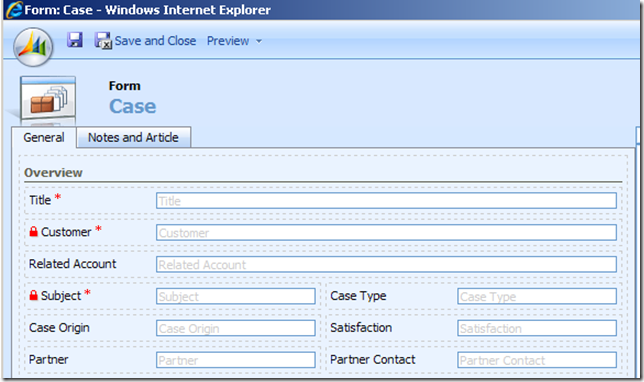
- While still on the Form, double-click the Customer field to open its details.
- On the Events tab, edit the OnChange event and insert this code:
//check to see that the customer is a contact, not account
if (crmForm.all.customerid.DataValue[0].typename = 'contact')
{
// Prepare variables for a contact to retrieve.
// Get the contact GUID
var contactid = crmForm.all.customerid.DataValue[0].id;
var authenticationHeader = GenerateAuthenticationHeader();
// Prepare the SOAP message.
var xml = "<?xml version='1.0' encoding='utf-8'?>"+
"<soap:Envelope xmlns:soap='http://schemas.xmlsoap.org/soap/envelope/'"+ " xmlns:xsi='http://www.w3.org/2001/XMLSchema-instance'"+ " xmlns:xsd='http://www.w3.org/2001/XMLSchema'>"+
authenticationHeader+
"<soap:Body>"+
"<Retrieve xmlns='http://schemas.microsoft.com/crm/2007/WebServices'>"+
"<entityName>contact</entityName>"+
"<id>"+contactid+"</id>"+
"<columnSet xmlns:q1='http://schemas.microsoft.com/crm/2006/Query' xsi:type='q1:ColumnSet'>"+
"<q1:Attributes>"+
"<q1:Attribute>parentcustomerid</q1:Attribute>"+
"</q1:Attributes>"+
"</columnSet>"+
"</Retrieve>"+
"</soap:Body>"+
"</soap:Envelope>";
// Prepare the xmlHttpObject and send the request.
var xHReq = new ActiveXObject("Msxml2.XMLHTTP");
xHReq.Open("POST", "/mscrmservices/2007/CrmService.asmx", false);
xHReq.setRequestHeader("SOAPAction","http://schemas.microsoft.com/crm/2007/WebServices/Retrieve"); xHReq.setRequestHeader("Content-Type", "text/xml; charset=utf-8");
xHReq.setRequestHeader("Content-Length", xml.length);
xHReq.send(xml);
// Capture the result.
var resultXml = xHReq.responseXML;
// Check for errors.
var errorCount = resultXml.selectNodes('//error').length;
if (errorCount != 0)
{
var msg = resultXml.selectSingleNode('//description').nodeTypedValue;
// alert(msg);
}
// Display the retrieved value.
else
{
//alert(resultXml.selectSingleNode("//q1:parentcustomerid").nodeTypedValue);
// this returns the Parent Account GUID
var accountid = resultXml.selectSingleNode("//q1:parentcustomerid").nodeTypedValue;
// }
//now get the account name using the accountid from above
// Prepare the SOAP message.
var xml = "<?xml version='1.0' encoding='utf-8'?>"+
"<soap:Envelope xmlns:soap='http://schemas.xmlsoap.org/soap/envelope/'"+ " xmlns:xsi='http://www.w3.org/2001/XMLSchema-instance'"+ " xmlns:xsd='http://www.w3.org/2001/XMLSchema'>"+
authenticationHeader+
"<soap:Body>"+
"<Retrieve xmlns='http://schemas.microsoft.com/crm/2007/WebServices'>"+
"<entityName>account</entityName>"+
"<id>"+accountid+"</id>"+
"<columnSet xmlns:q1='http://schemas.microsoft.com/crm/2006/Query' xsi:type='q1:ColumnSet'>"+
"<q1:Attributes>"+
"<q1:Attribute>name</q1:Attribute>"+
"</q1:Attributes>"+
"</columnSet>"+
"</Retrieve>"+
"</soap:Body>"+
"</soap:Envelope>";
// Prepare the xmlHttpObject and send the request.
var xHReq = new ActiveXObject("Msxml2.XMLHTTP");
xHReq.Open("POST", "/mscrmservices/2007/CrmService.asmx", false);
xHReq.setRequestHeader("SOAPAction","http://schemas.microsoft.com/crm/2007/WebServices/Retrieve"); xHReq.setRequestHeader("Content-Type", "text/xml; charset=utf-8");
xHReq.setRequestHeader("Content-Length", xml.length);
xHReq.send(xml);
// Capture the result.
var resultXml = xHReq.responseXML;
// Check for errors.
var errorCount = resultXml.selectNodes('//error').length;
if (errorCount != 0)
{
var msg = resultXml.selectSingleNode('//description').nodeTypedValue;
// alert(msg);
}
// Display the retrieved value.
else
{
//alert(resultXml.selectSingleNode("//q1:name").nodeTypedValue);
//var accountid = resultXml.selectSingleNode("//q1:parentcustomerid").nodeTypedValue;
// set the related account value
crmForm.all.case_relatedaccount.DataValue = resultXml.selectSingleNode("//q1:name").nodeTypedValue;
}
// close the else from getting the parentcustomerid
}
// close the main if statement when checking for typename
}
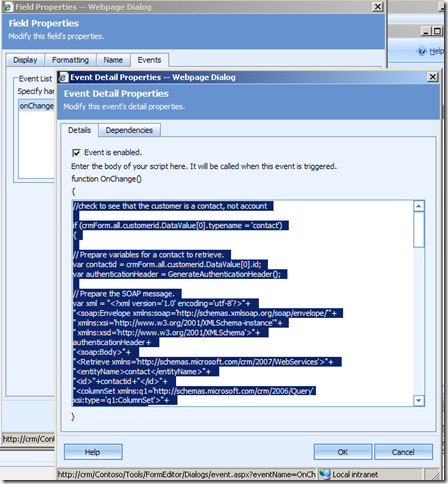
Save the changes and publish and test! It should fill in the Related Account field with the contact’s parent customer name.
I still need to implement some more checking on things, but it does work as intended.